HTTPSorHTTP
The Illusion of Web Security: A Cautionary Tale
Alex was a bright developer. Fresh out of college and eager to make a mark, he landed a job at a promising tech startup. His first major project: build a new web application for the company’s clients. As Alex dove into the world of code, he kept hearing the same advice from his peers and mentors: “Make sure the site uses HTTPS. It’s the gold standard for web security.”
Alex took this to heart. From day one, he made HTTPS his mission. He knew it would protect the site from snooping and ensure that the data transmitted between the user’s browser and the server would be encrypted. With the famous padlock icon in the browser’s address bar, everything would be safe. Right?
His hard work paid off. After weeks of coding, testing, and polishing, the new web app went live. It was slick, fast, and—most importantly—secured with HTTPS. Alex proudly showed it to his manager, who was impressed by his attention to detail. The clients, too, were happy, particularly with the sense of security that came with the encrypted connection. The padlock symbol was a badge of trust.
But Alex’s sense of accomplishment didn’t last long.
The First Crack
One evening, Alex received an urgent message from the support team: a customer had reported that their account had been hacked. Alex’s heart skipped a beat. He immediately dismissed it as user error. Perhaps the customer had used a weak password, or maybe they’d been careless with their login credentials.
However, over the next few days, more complaints started rolling in. Customers claimed their accounts were compromised despite the web app showing the padlock in the browser. Confused and frustrated, Alex decided to investigate.
Upon digging deeper, he found something peculiar—one of the compromised accounts had been accessed from an HTTPS-secured site that wasn’t even part of their company’s domain. Yet, to the customer, it had looked identical to the legitimate site, complete with the padlock. It was a classic phishing attack.
“How could this happen?” Alex thought. “We use HTTPS! The data is encrypted!”
What Alex didn’t realize was that HTTPS only encrypted the data between the browser and the server. It didn’t prevent an attacker from creating a clone of his site, complete with a legitimate SSL certificate, tricking users into submitting their sensitive data. The padlock didn’t mean the site was safe—it just meant the connection was encrypted.
The Security Audit
Still reeling from the phishing debacle, the company hired an external security auditor to assess their systems. Alex nervously sat in on the first meeting. The auditor was a grizzled, no-nonsense professional who had seen it all.
“HTTPS is a good start,” the auditor began. “But it’s just one piece of the puzzle.”
Alex felt his stomach tighten.
The audit was thorough, and the findings were shocking. The auditor identified several vulnerabilities that HTTPS had done nothing to protect against.
1. SQL Injection
The first vulnerability was classic SQL Injection. The auditor demonstrated how a seemingly innocent input field in the app’s login form could be exploited to extract user data from the database.
Alex had used the following code to check a user’s credentials in the database:
|
|
The auditor typed in admin' OR '1'='1
into the username field. To Alex’s horror, the app logged him in without the need for a password. The SQL query was manipulated by the attacker’s input, bypassing authentication entirely.
The auditor explained that Alex needed to use prepared statements and parameterized queries to protect against this:
|
|
The difference was staggering—this small change would ensure that user inputs couldn’t interfere with the SQL query, keeping the database safe from injection attacks. HTTPS had done nothing to prevent this flaw.
2. Cross-Site Scripting (XSS)
Next came Cross-Site Scripting (XSS). The auditor pointed out that some of the web app’s forms allowed users to input HTML and JavaScript without proper validation. This opened the door for attackers to inject malicious scripts directly into the application.
Alex had built a simple comment section, but he hadn’t sanitized user inputs properly:
|
|
An attacker could easily inject a script like this:
|
|
As soon as the page loaded, the malicious script would execute, hijacking the user’s session or stealing their credentials. Again, HTTPS couldn’t stop this attack—it only encrypted the communication.
To fix this, the auditor showed Alex how to sanitize user inputs by escaping HTML characters:
|
|
This would ensure that any script tags or special characters were rendered harmless by converting them into plain text.
3. Security Misconfiguration
The auditor also uncovered several security misconfigurations that had left the application vulnerable. For instance, Alex had exposed an administrative panel to the internet with the default username and password still in place.
|
|
The auditor shook his head. “This is a ticking time bomb,” he said. “Anyone could brute-force their way into the admin panel.”
The solution was clear: Alex needed to secure these sensitive endpoints by enforcing strong, unique passwords, implementing multi-factor authentication, and limiting access by IP.
The Final Blow: Mixed Content
As Alex continued to learn about the flaws in his application, the auditor dropped one more bombshell. Some parts of the web app—like images and scripts—were being served over HTTP instead of HTTPS. This mixed content left the site vulnerable to man-in-the-middle attacks.
An attacker could intercept and modify these HTTP resources, injecting malicious code into the page even though the main connection was secured by HTTPS.
Alex had assumed that the padlock meant everything was secure, but this wasn’t true. The auditor showed him how to ensure all resources were served over HTTPS:
|
|
He also had to enable strict HTTPS enforcement in the app’s configuration.
The Fallout
The next few weeks were brutal for Alex. He worked around the clock with the security team, patching vulnerabilities, fixing configurations, and implementing stricter input validation. He hardened the authentication mechanisms, ensured proper escaping of all user input, and enforced HTTPS across the board, leaving no room for mixed content issues.
As he worked, Alex couldn’t shake the feeling that he had been lulled into a false sense of security by HTTPS. He had thought that once encryption was in place, his job was done. He had assumed that the padlock in the browser was the ultimate symbol of safety.
But it wasn’t. And it never had been.
The Lesson
Alex learned a hard but valuable lesson: HTTPS is crucial, but it’s far from sufficient. It protects the transmission of data between the browser and the server, but it doesn’t stop attacks that target the application itself. Security was not a single layer, like a wall or a padlock. It was a multi-faceted, constantly evolving discipline.
The web is full of dangers that HTTPS alone cannot defend against: phishing, social engineering, injection attacks, cross-site scripting, broken authentication, and more. The illusion that HTTPS guarantees complete security is just that—an illusion. Like France’s Maginot Line, it can be bypassed, leaving you vulnerable if you don’t plan for the broader threats.
Alex made a promise to himself: he would never again make the mistake of assuming that one security measure was enough. Web security was about layers, vigilance, and always staying one step ahead of the attackers.
And now, when people asked him if HTTPS was enough, he had a simple answer:
“It’s just the beginning.”
Related Articles:
- 2015/05/30 https site available now with cert from cacert.org
- 2010/03/09 swaks – Swiff army nife for SMTP
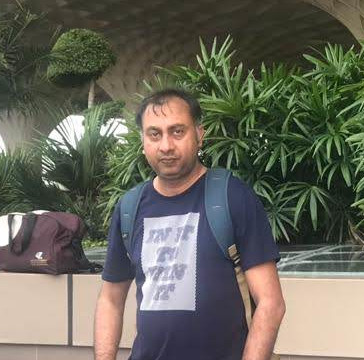
Authored By Amit Agarwal
Amit Agarwal, Linux and Photography are my hobbies.Creative Commons Attribution 4.0 International License.